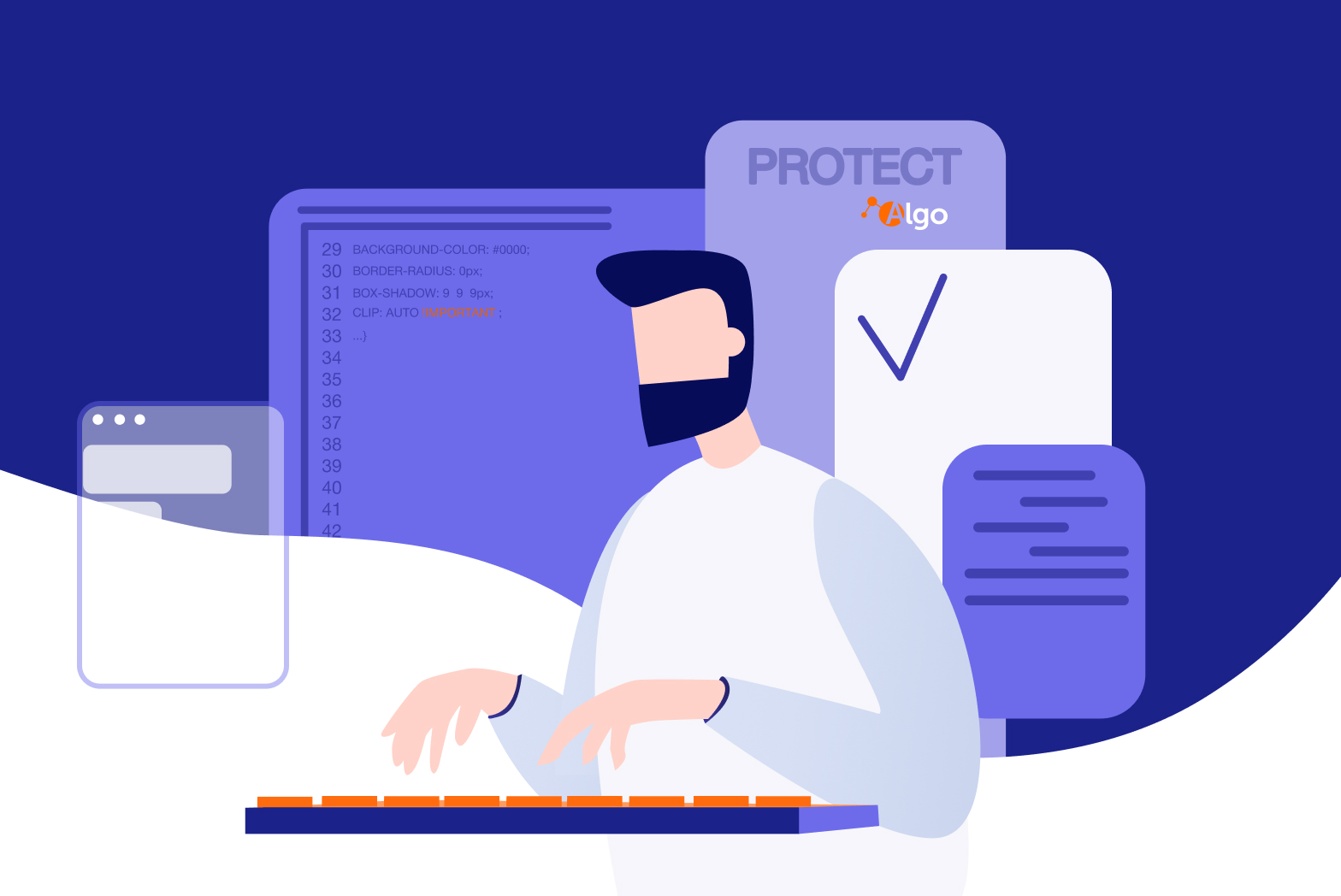
CSS or Cascade Sheet Styling is one of the main components in website designing. If HTML is the skeleton or structure of a web page, CSS is its presentation. It helps in building a visually appealing website. However, the use of CSS with HTML and JavaScript can get a bit tricky. There are some conventions and rules to follow. These rules make the CSS designing process universal and easy to apply. In this article, we will define some of the concepts which are the starting point, and hence, are one of the building blocks of CSS.
Inheritance
The concept of inheritance is of primary importance in many programming languages. Through inheritance, the child elements adopt the functionalities from the parent elements. There is a concept of overriding where the child has their own properties. They do not adopt the property from their parents, like in a real-world inheritance. Let’s understand the concept through an example.
<!DOCTYPE html>
<html>
<head>
<style type="text/css">
#parentdiv {
font-style: normal;
font-size: 30px;
border: 5px solid blue;
font-family: monospace, sans-serif;
}
</style>
</head>
<body>
<div id="parentdiv">
<p>This is a parent element.</p>
<div class="firstchild">
<p>I am the first child.</p>
</div>
<div class="secondchild">
<p>I am the second child.</p>
</div>
</div>
</body>
</html>
We created a div element as a parent containing two other div elements. In the style tag, we defined our CSS rules. Notice the parent div styling is applied to its child elements as well. Although we did not specifically mention CSS styling for the child elements, they adopted the parent element’s styling due to inheritance. It is useful, especially when we design large websites. Also, the parent and their child do not need to be the same elements. We can also place paragraph elements in the parent div and get the same CSS applied to it. This is possible due to inheritance.
One property to note in the output is of the border. The parent div gets a blue border but not the child div elements. It indicates that not all properties are inherited. Usually, the child elements do not inherit the position and background properties.
Some of the values for the inheritance for an element are:
Inherit which sets the value of the property as the parent element value.
Initial which sets the value to initial or default element value.
Unset which either sets the value to the inherited value if it is naturally inherited or resets to the initial value.
Revert which rollbacks the value to the one before any changes. It could be either inherit or initial. It is a fairly new property, and some browsers may not support it.
Cascade
What is cascade in a cascade sheet style? In CSS, more than one styling rule can be applied to an element. When this happens, the rules are cascaded, and the last one is picked. In simple words, it is the rule that applies directly to the element and is in the last. We have seen in the above example that sometimes we do not apply styles to an element, and still, it gets styled due to inheritance. But what happens if we have multiple styles for the same element? Let’s see another example.
<!DOCTYPE html>
<html>
<head>
<style type="text/css">
div {
font-style: oblique;
font-size: 30px;
border-width: 5px;
font-family: monospace, sans-serif;
border: solid blue;
}
.div1{
border: dotted red;
}
</style>
</head>
<body>
<div>
<p>I am the first div.</p>
</div>
<div>
<p>I am the second div.</p>
</div>
<div class="div1">
<p>I have my own styling</p>
</div>
</body>
</html>
Here, all the divs are styled using the CSS defined for a div. For the third div, we see the class selector overrides the div styles. If we add another div styling after this one, the new styles apply to the first two divs. This is cascading. The styling of the third div is still vague. Move .div1 to the top. It still works. Why? According to the cascade rule, the last one should work. It brings us to another important concept, which is Specificity.
Specificity
When multiple CSS rules apply to an element, the rule with the highest specificity is selected. The elements are selected by type name, class name, and id. The least specific is the element type selector, and the highest specific is the id selector. In the example, the class selector of the third div is selected for styling as it is the most specific.
More than one element can be assigned the same class name. If we have three elements with the same class and we want to style one of them differently, we can assign it an id. The id selector will be used for its styling as it is the most specific.
<!DOCTYPE html>
<html>
<head>
<style type="text/css">
div {
font-style: oblique;
font-size: 30px;
border-width: 5px;
font-family: monospace, sans-serif;
border: solid blue;
}
.div1{
font-weight: initial;
border: dotted red;
}
#diffdiv{
font-weight: bold;
}
</style>
</head>
<body>
<div>
<p>I am the first div.</p>
</div>
<div>
<p>I am the second div.</p>
</div>
<div class="div1">
<p>I have my own styling</p>
</div>
<div id="diffdiv" class="div1">
<p>I am a different div.</p>
</div>
</body>
</html>
If two style rules are there for an element with the same specificity, then the one in the end is applied. This rule is also known as source order.
!important
This special rule overrides all the rules discussed above. Therefore, it should be used with care. If we use !important, it means this specific style rule applies no matter its position in the cascading hierarchy and the specificity score.
As it overrules the normal CSS rules, it is advised against the use of !important in styling. It may make the debugging of CSS code difficult, especially in the case of large style sheets. One should use it only if it is extremely needed. One of its uses is when there is no access to the core CSS module in the software. In this case, we can override the rules using !important declarations. CMS software often poses such situations to the CMS managers.
The following example explains this concept.
<!DOCTYPE html>
<html>
<head>
<style type="text/css">
div {
font-style: oblique;
font-size: 30px;
border-width: 5px;
font-family: monospace, sans-serif;
border: solid blue;
}
.div1{
border: none !important;
}
#diffdiv{
font-weight: bold;
border: dotted red;
}
</style>
</head>
<body>
<div>
<p>I am the first div.</p>
</div>
<div class="div1">
<p>I have my own styling</p>
</div>
<div id="diffdiv" class="div1">
<p>I have my own styling</p>
</div>
</body>
</html>
There are three style rules for a div. First is the element selector with the least specificity. It styles the div with a blue solid border. The second style is of the class selector that specifies the border as none. The third is the id selector with the highest specificity. It defines a dotted red border. According to the normal CSS cascade and specificity rule, the id selector should be given preference, and the div with the id diffdiv should have a dotted red border. But in our example, !important declaration in the class selector overrides this rule, and the two divs with class div1 style with the border: none. Like this,
Inheritance, Cascade, and Specificity together control the styling of HTML elements on a web page. As the length of code increases, these concepts can get a bit tricky. Understanding these basic concepts of CSS lays the foundation for understanding more complex concepts in web designing.