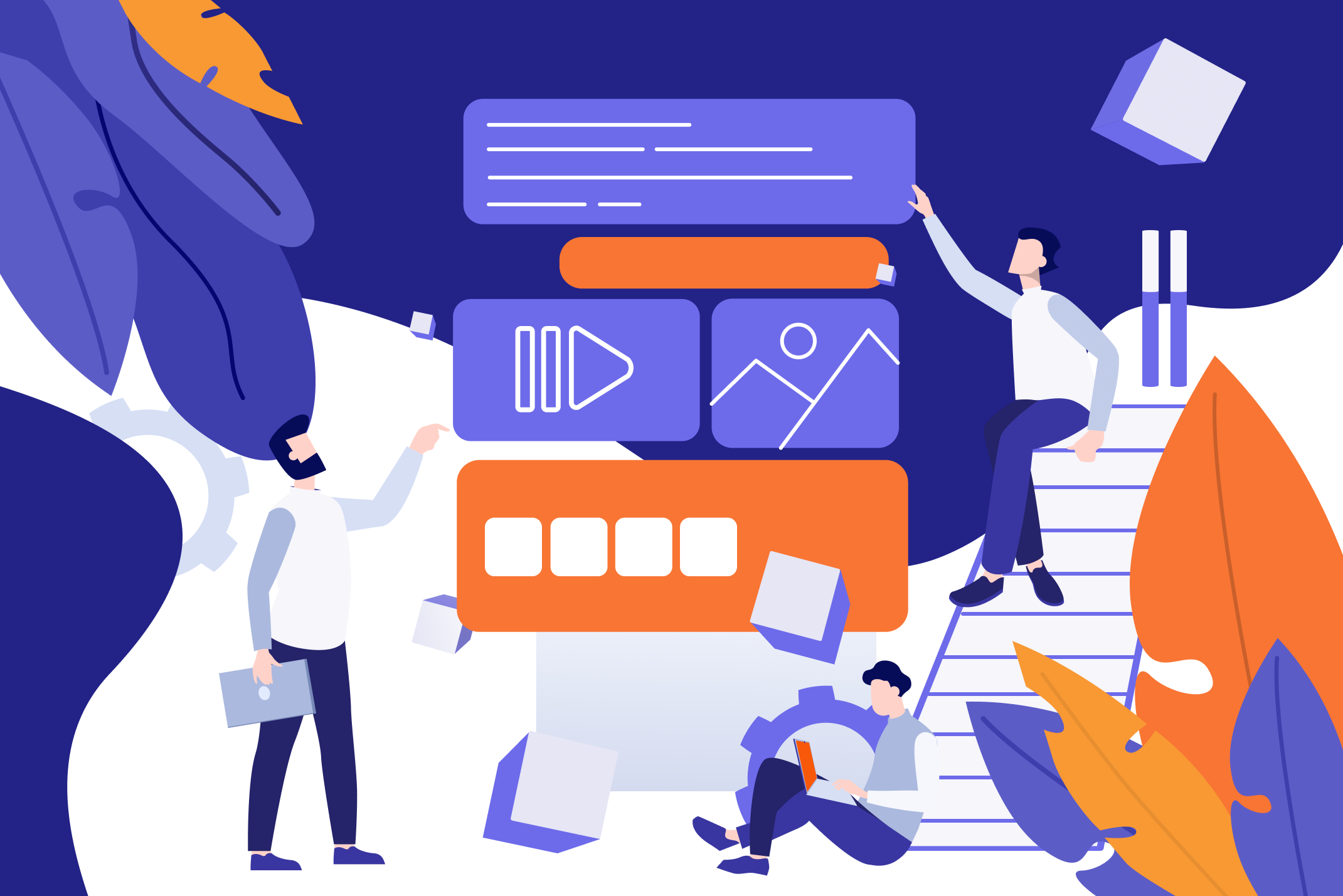
The box model in CSS defines where every element gets placed relative to all of the other elements on a webpage. The final rendering of a webpage is made according to the box model. With a large webpage and its elements, the desired final look of every element relative to other elements can become confusing to determine. The box model helps maintain some ground rules and makes the overall styling easier for the web designer. Hence, it is one of the main building blocks of CSS.
The Box Model
The box model sees every HTML element on a webpage as a box. There are some types of boxes and some associated properties. If we write a <p> tag in our HTML document and open the file in a browser, we see the p tag as a text. To get a clear picture, we will apply a background-color style to it. We will see the <p> tag as a box.
<!DOCTYPE html>
<html>
<head>
<style type="text/css">
p{
background-color: lightgreen;
}
</style>
</head>
<body>
<div>
<p>I am a box.
</p>
</div>
</body>
</html>
Types of Boxes in CSS
We deal with two types of boxes normally, block and inline. There are some elements with the default display as a block, like div and p tags. Some elements are inline, such as a span element. Span, by default, has inline as a display value.
Each box display has the following properties
- Content: the inner HTML part of an HTML element forms its content. In the case of <img> the entire image is the content. With <li> the text inside the list tags is the inner html or content.
- Padding: the space around the content of an element. The padding has the same background color as the content.
- Border: the space around the padding which visibly separates an element from the surrounding. Different styles are applied to an element border to make it distinct.
- Margin: margin invisibly separates HTML elements from one another. The white space between the elements is due to margin space.
Block display
Let’s look at an example to see how a block display and its properties work.
<!DOCTYPE html>
<html>
<head>
<style type="text/css">
div p {
padding: 5px;
border: solid darkblue;
margin: 5px;
}
</style>
</head>
<body>
<div>
<p>I have a box display.
</p>
</div>
</body>
</html>
If we right-click on the webpage, select inspect, we can see the box model on the right-hand side. Even if we do not set the display as a block, it sets as a block by default. Also note, for padding and margin, we can define different values for all four sides of an element through margin-top, margin-bottom, margin-left, margin-right. The same goes for the padding.
The dark blue border around the div shows that the block display stretches over all of the available container space. It extends in an inline manner that is horizontal. If we place another element, it will shift to the next line on the webpage. Also, the width and height properties can be set in the block element. Height and width make the block display according to the developer’s preference instead of stretching 100 percent. We can go ahead and this by adding a height of 10px and a width of 20px in the above styling. The display changes a lot!
Inline Display
Now, let’s see how an inline display differs from a block display. We will also define a height for our div and p elements. Note, by default <p> has block style but we are making <p> display as ‘inline’ here.
<!DOCTYPE html>
<html>
<head>
<style type="text/css">
div p {
padding: 5px;
border: solid darkblue;
margin: 5px;
display: inline;
height: 500px;
}
</style>
</head>
<body>
<div>
<p>I have an inline display.
</p>
<p>
Me too.
</p>
</div>
</body>
</html>
We notice that there is no line break. The two <p> elements are next to each other in a line. Also, what about the height=500px? The p elements are much shorter than this. The reason for this is that the inline display does not set the height and width of the element. So, if we have an inline element, we have no control over its height and width as in a block display.
The inline display also gets a bit tricky when it comes to margins and padding. In the above example, we see the two p elements have a space between them. Margin: 5px and padding: 5px sets margin and padding values of 5px on all four sides. But when we inspect the elements, top and bottom margins are not showing (marked in orange in the image below). It shows that an inline display does not consider vertical margins and padding while considering horizontal margin and padding. We will get the idea more clearly in the next example.
Now, we will display another p element in the next line and see the results.
<!DOCTYPE html>
<html>
<head>
<style type="text/css">
p {
padding: 5px;
border: solid darkblue;
margin: 5px;
display: inline;
height: 500px;
}
</style>
</head>
<body>
<div>
<p>I have an inline display.
</p>
<p>
Me too.
</p>
</div>
<div>
<p>I am on a next line.</p>
</div>
</bod
The output display clearly shows that vertical margins and padding are not respected. The p elements are overlapping with no distance in between.
Inline-Block Display
With block display, the elements span over all of the available space, whereas, with inline, there are vertical spacing issues and no control over height and width. Inline-block is an in-between solution. It aligns elements with no line break while maintaining the vertical and horizontal margins and padding. It also considers the height and width of an element, unlike an inline display. We can change the display value to inline-block in the above style definition and see the difference.
Now, the margin and padding values are set on all four sides. Moreover, the height of p elements is set to 500px.
Inner Display
The block and inline displays are known as the ‘outer’ display for an element. In CSS, there is also the concept of the inner display. It defines how the elements inside a block or an inline element will display on a page. The inner display can be a flex display or a grid display. Inner display is worth mentioning because a web browser considers block and inline as ‘normal’ display types, and there are other ways to play with the display of elements within the elements. If any of such inner displays are not mentioned, the browser will treat block and inline as a normal flow or a display of elements.
<!DOCTYPE html>
<html>
<head>
<style type="text/css">
input {
padding: 5px;
border: solid darkblue;
margin: 5px;
display: flex;
}
</style>
</head>
<body>
<form id="form">
<input type="text" placeholder="username">
<input type="password" placeholder="password">
<input type="button" value="OK">
</form>
</body>
</html>
By default, the form element has a block display, and inputs have an inline display. By setting the input display property to flex, we tell the browser to overrule the normal inner display for the form element and follow the flex rules.
Border-Box
Let’s take a look at one last point before ending the box model discussion. In the standard practice, we add margins and padding in addition to the height and width of an element, like we saw in the above examples on box display. So, when we are figuring out the space that an element will take up on a webpage, we have to take into account the associated padding and margins. This practice is not very convenient, especially when one is designing a large website. An alternate solution to this is the box-sizing property.
Box-sizing = border-box considers the padding and border of an element included in its width and height. So, the width and height of the content are the width and height minus the padding and border values. Note, the margin is not included in this. The margin defines the outer space of an element, its distance from the surrounding.
<!DOCTYPE html>
<html>
<head>
<style type="text/css">
p {
box-sizing: border-box;
width: 250px;
height: 100px;
margin: 20px;
padding: 10px;
border: dotted green;
}
</style>
</head>
<body>
<div>
<p>I have box-sizing set as border-box.
</p>
</div>
</body>
</html>
This sets the content width to 224px. 250px minus 10 (padding-left) minus 10 (padding-right) minus 3 (default border width on left) minus 3 (default border width on right). Similarly, height is 100px minus padding and border on top and bottom, equals 74.
To see how it is different from the standard box model, we can remove the box-sizing property. The width and height of the content will be 250px and 100px, respectively.
With this, we end the box model discussion. It is a basic concept regarding the placement and flow of elements related to one another on a webpage.
Algo is a one-click solution for developers and designers to secure authorship over their source code, design or screenshots via blockchain technologies. To learn more visit https://algo.id